はじめに
discord.js
の勉強のために作った,OpenAI 社提供の LLM を使った Discord Bot です.
ソースコードは,以下の GitHub にあります.
実装方法についてはリポジトリ内のソースコードや README.md
を参照してもらうとして,この記事では Discord Bot を実装して思ったこととか,短期間運用してみての感想とかを書いていきます.
ちなみに,GitHub には Anthropic-Bot という Anthropic 社の LLM を使った Discord Bot もありますが,実装上の考え方は全く同じです(LLM ごとの回答性能を比較したかったので).
最終形
flowchart LR
style User stroke:#3465A4,stroke-width:2.5px,fill:#EAEFF5,color:#09150A
style Discord stroke:#09150A,stroke-width:2px,fill:#7289DA
style Server stroke:#CC0000,stroke-width:2.5px,fill:#F9E5E5,color:#09150A
style OpenAI-Bot stroke:#09150A,stroke-width:2px,fill:#26000B
style External stroke:#74AA9C,stroke-width:2.5px,fill:#E6F4F1,color:#09150A
style bot stroke:#09150A,stroke-width:2px,fill:#FFF3E0,color:#09150A
style utils stroke:#09150A,stroke-width:2px,fill:#E8F5E9,color:#09150A
style commands stroke:#09150A,stroke-width:2px,fill:#E1F5FE,color:#09150A
subgraph User
direction LR
subgraph Discord["Client Discord"]
direction LR
Chat("Slash Command")
end
end
subgraph Server
direction LR
subgraph OpenAI-Bot["OpenAI-Bot (npm)"]
direction LR
subgraph bot
direction LR
index(index.js)
end
subgraph commands
direction LR
chat(chat.js)
image(image.js)
translate(translate.js)
Help(help.js)
end
subgraph utils
direction LR
logger(logger.js)
messenger(messenger.js)
end
end
end
subgraph External
direction LR
OpenAI(("OpenAI API"))
end
Chat -->|"<strong>1.</strong> Command Execution"| bot
index -->|"<strong>2.</strong> Command Handler"| commands <-->|<strong>3.</strong> API Execution / Response| OpenAI
commands <-->|"(Common Functions)"| utils
commands -->|"<strong>4.</strong> Command Response"| Discord
linkStyle 0 stroke:#7289DA,stroke-width:2px
linkStyle 2 stroke:#75507B,stroke-width:2px
linkStyle 4 stroke:#7289DA,stroke-width:2px
実装上のポイント
実装ファイルの分割
これは生成 AI とか Discord Bot に限らず大抵の実装全般に言えることですが,処理ごとにファイルを分割して実装することでメンテナンス性が向上します.
コマンドの実装
ファサードになる index.js
の DISCORD.once('ready', async () => { ... })
内でコマンドを読み込む処理を行い,commands
オブジェクトにコマンド名をキーとしてコマンドのデータを格納しています.
// Bot が起動したときの処理
DISCORD.once("ready", async () => {
// コマンドを読み込む
await loadCommands();
// コマンドを登録
const data = [];
for (const commandName in commands) {
data.push(commands[commandName].data);
}
await DISCORD.application.commands.set(data);
await logger.logToFile(`${DISCORD.user.tag} でログインしました`);
});
// `../commands` ディレクトリ内のコマンドを読み込む
async function loadCommands() {
const commandFiles = await FS.readdir(PATH.resolve(__dirname, "../commands"));
const jsFiles = commandFiles.filter((file) => file.endsWith(".js"));
for (const file of jsFiles) {
const command = require(PATH.resolve(__dirname, `../commands/${file}`));
commands[command.data.name] = command;
await logger.logToFile(
`コマンド \`${command.data.name}\` を読み込みました`
);
}
}
新しいコマンドを増やすときや既存のコマンドを削除するときは,commands
ディレクトリ内のファイルごと追加・削除するだけで他のコマンドに影響を与えることなく実装できます.
非機能周りの処理
ロギングやメッセージ送信などの非機能周りの処理は,コマンド実装ファイルから分離して utils
ディレクトリ内に格納しています.
これによりコマンド実装ファイルはコマンドの処理に集中でき,それ以外は共通関数を呼び出すだけで実装できます.
コミュニティサーバでの運用を想定して
今回作った Bot は個人的に使用していますが,コミュニティサーバでの運用も想定して実装しています.
重要になるのはコスト管理で,AI の従量課金はトークン数で決まるモデルがほとんどのため,logger.js
でログを取りながらコストを把握できるようにしています.
// コマンドを起動したユーザ情報をファイルにのみ書き込む
commandToFile: async function (interaction) {
const logFilePath = getLogFilePath('openai-bot.log');
const userInfo = [
`---------- ユーザ情報 ----------`,
`コマンド : ${interaction.commandName}`,
`ユーザ名 : ${interaction.user.username}`,
`ユーザID : ${interaction.user.id}`,
`--------------------------------`
].join('\n');
await FS.appendFile(logFilePath, `\n\n${userInfo}\n`);
},
// コマンド実行で使用したトークンをファイルに書き込む
tokenToFile: async function (usedModel, usage) {
const logFilePath = getLogFilePath('openai-bot.log');
const tokenInfo = [
`---------- モデル情報 ----------`,
`使用モデル : ${usedModel}`,
`--------- トークン情報 ---------`,
`質問トークン : ${usage.prompt_tokens}`,
`回答トークン : ${usage.completion_tokens}`,
`総計トークン : ${usage.total_tokens}`,
`--------------------------------`
].join('\n');
await FS.appendFile(logFilePath, `${tokenInfo}\n`);
},
最新の実装は GitHub のコードを参照してください.
一応,使用モデルごとにトークン数 × 単位料金でユーザごとのコストも自動計算できますが,今はそこまでは実装していません.
コマンドごとの使用モデルの切り替え
OpenAI API はモデルごとにトークン単位料金や回答品質が異なるため,コマンドごとに使用モデルを切り替えられるようにしています.
特に chat.js
では,基本的には安くて速い gpt-4o
を使っていますが,コード生成系のコマンドでは o3-mini
を使っています.
o1
は性能はいいものの料金がかかるモデルなので,特に理由がない限りは使わないようにしています.
// プロンプトタイプに応じたモデルの選択
const codePrompts = ["code", "code_analysis", "code_review", "log_analysis"];
let modelToUse;
if (promptParam === "reasoning") {
modelToUse = "o1";
} else if (codePrompts.includes(promptParam)) {
modelToUse = "o3-mini";
} else {
modelToUse = "gpt-4o";
}
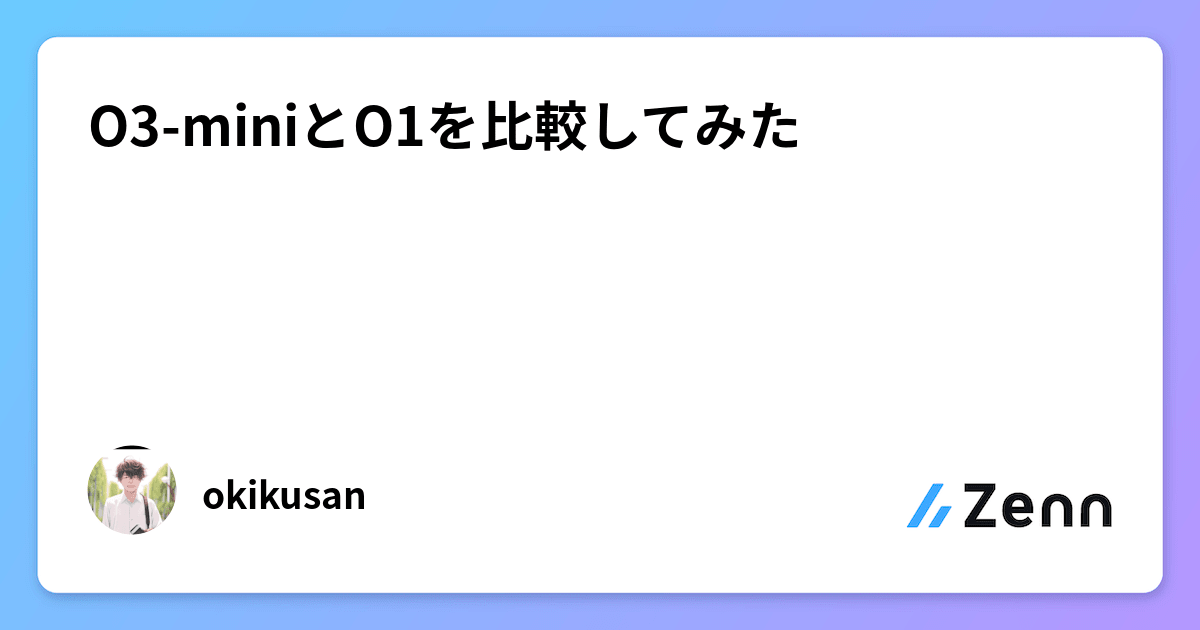
長文入力補助
Discord の Slash Command 入力欄は改行が使えなかったり Markdown のプレビューができなかったりするため,長文入力の補助機能として添付ファイルを受け付けるようにしています.
コード生成系のコマンドで長文を入力する際には,.md
ファイルなどを添付してもらうことで指示文と添付ファイルの内容を組み合わせて AI に問い合わせることができます.
// 添付ファイルがある場合は内容を取得
let attachmentContent = "";
if (interaction.options.get("添付ファイル")) {
const attachment = interaction.options.getAttachment("添付ファイル");
// 添付ファイルがテキストの場合は質問文に追加
if (attachment.contentType.startsWith("text/")) {
try {
const response = await fetch(attachment.url);
const arrayBuffer = await response.arrayBuffer();
attachmentContent = Buffer.from(arrayBuffer).toString();
} catch (error) {
await logger.errorToFile("添付ファイルの取得中にエラーが発生", error);
}
}
await logger.logToFileForAttachment(attachmentContent.trim());
}
過去ログの参照
世の中の AI チャットツールは,過去の会話ログも参照して回答を返すことができるものが多いです.
が,Discord Bot は複数人がチャットする中で機能するもののため,直前のチャットが AI に渡す過去ログになるとは限りません.
そこで,コマンド使用ユーザごとに過去ログをファイルに保存しておき,過去ログを参照する場合にはそのファイルを読み込んで AI に渡すようにしています.
chat.js
での過去ログの参照は以下のようになります.
// 直前の会話を利用する場合
let previousQA = "";
if (usePrevious) {
try {
previousQA = await logger.answerFromFile(userId);
await logger.logToFile(`前回 : ${previousQA.trim()}`);
} catch (error) {
await logger.errorToFile("直前の会話の取得でエラーが発生", error);
}
}
直前の会話を logger.js
でファイルから読み込む処理は以下のようになります.
// 直前の会話をファイルから読み込む
answerFromFile: async function (userid) {
const logFilePath = getLogFilePath(`openai-bot-${userid}.log`);
let previousQA = '';
try {
previousQA = await FS.readFile(logFilePath, 'utf-8');
} catch (error) {
if (error.code !== 'ENOENT') throw error;
}
return previousQA;
},
(現状の)生成 AI でできなかったこと
2025 年時点での o1
や o3-mini
,gpt-4o
での評価です.
生成後の回答文字数は制御できない
文章生成 AI の特性を考えれば当たり前なのですが,AI は回答文をリアルタイムで(思考して)書いている訳では無いです.
実際には「過去大量の学習データに基づくと,こういう文章に続くのはこういう文章だろう」という確率的な予測を行っているのが近いと思っています.
で,何が問題だったかというと「回答文の文字数を制御できない」ことです.
discord.js
で slash command
を使っているのですが,slash command
は 2000 文字までしか返せない仕様になっています.
Field | Type | Description |
---|---|---|
content?* | string | Message contents (up to 2000 characters) |
ソースコード生成など長文のレスポンスを返してほしい場合,大抵は 2000 文字を超えてコンソールに以下のエラーが表示されます.
DiscordAPIError: Invalid Form Body
content: Must be 2000 or fewer in length.
単純に system
などの強い メッセージの役割(messages.role
) 使って「2000 文字を超える場合は分割して送信する」などの制御を試みても,まったく文字数カウントがされないままレスポンスが返ってきてエラーになります.
最終的に,自分はこういった「文章生成」以外の処理は一切 AI に任せず,実装側の JavaScript で制御することにしました.
文章生成 AI の回答フォーマットは Markdown になることがほとんどなので,それを考慮して回答文を分割する関数を実装しました.
function splitAnswer(answer) {
const maxLen = 1900; // Discord の文字数制限に余裕をもたせる
let messages = [];
let currentMessage = "";
let inCodeBlock = false;
let codeLanguage = "";
const lines = answer.split("\n");
for (const line of lines) {
// コードブロックの開始または終了を検出
if (line.startsWith("```")) {
// コードブロックの開始地点
if (!inCodeBlock) {
inCodeBlock = true;
codeLanguage = line.slice(3).trim();
if (currentMessage.length + line.length > maxLen) {
messages.push(currentMessage.trim());
currentMessage = "";
}
currentMessage += line + "\n";
}
// コードブロックの終了地点
else {
inCodeBlock = false;
currentMessage += line + "\n";
if (currentMessage.length > maxLen) {
messages.push(currentMessage.trim());
currentMessage = "```" + codeLanguage + "\n";
}
}
}
// コードブロック内の処理
else if (inCodeBlock) {
// コードブロック内で分割する場合、閉じタグと開始タグを追加
if (currentMessage.length + line.length > maxLen) {
currentMessage += "```\n";
messages.push(currentMessage.trim());
currentMessage = "```" + codeLanguage + "\n" + line + "\n";
} else {
currentMessage += line + "\n";
}
}
// 通常のメッセージ処理
else {
// 最大長を超える場合に新しいメッセージを開始
if (currentMessage.length + line.length > maxLen) {
messages.push(currentMessage.trim());
currentMessage = line + "\n";
} else {
currentMessage += line + "\n";
}
}
}
// 残りのメッセージを追加
if (currentMessage.length > 0) {
messages.push(currentMessage.trim());
}
return messages;
}
Discord への返答は messages
配列に格納された文字列を順番に送信することで,2000 文字を超える回答文でもエラーなく送信できるようになります.
// 回答を分割
const splitMessages = splitAnswer(answer.message.content);
// 単一メッセージの場合
if (splitMessages.length === 1) {
await interaction.editReply({
content: messenger.answerMessages(openAiEmoji, splitMessages[0]),
flags: isPublic ? 0 : MessageFlags.Ephemeral,
});
}
// 複数メッセージの場合
else {
for (let i = 0; i < splitMessages.length; i++) {
const message = splitMessages[i];
if (i === 0) {
await interaction.editReply({
content: messenger.answerFollowMessages(
openAiEmoji,
message,
i + 1,
splitMessages.length
),
flags: isPublic ? 0 : MessageFlags.Ephemeral,
});
} else {
await interaction.followUp({
content: messenger.answerFollowMessages(
openAiEmoji,
message,
i + 1,
splitMessages.length
),
flags: isPublic ? 0 : MessageFlags.Ephemeral,
});
}
}
}
最新の実装は GitHub のコードを参照してください.
埋め込みプロンプトの最適化
生成文章の振る舞いを制御したい場合は,大抵 system
ロールや developer
ロールで指示を(裏で)埋め込んでから,ようやくユーザからの指示文を user
ロールで受け付けるという流れになると思います.
この埋め込みプロンプトは詳しく書けば書くほど,AI の回答は的確に,かつ安定して同一クオリティの回答を返してくれます.
が,毎回呼び出される定型文のため,やりすぎると 1 回のリクエストごとのトークン数が増えてしまい,かなり加速度的に API の使用量が増えてしまいます.
プロンプトテクニック的に言うと,基本的には Zero-shot で埋め込みプロンプトは最小限に抑えておき,ブレてほしくないコマンド部分にのみ Few-shot で前提や回答例を詳しく埋め込むというのが良いと思います.
今回作った Bot の場合は,決まったフォーマットの Git コミットメッセージを生成するコマンドでは,Few-shot で埋め込みプロンプトを最適化しています.
case 'commit':
return `ユーザからの「変更内容」に対して,Git のコミットメッセージのヘッダ(1行目)を \`:emoji: prefix / Subject\` のテンプレートで箇条書きで 3 候補作成してください.1 行目には「変更内容」のみを英文で簡潔にまとめて,2 行目以降から箇条書きで表示してください.
emoji は次の中から選択してください.\`:bug:\` for Bug fixes, \`:+1:\` for Feature improvement, \`:sparkles:\` for Partial function addition, \`:tada:\` for A big feature addition to celebrate, \`:art:\` for Design change only, \`:shirt:\` for Lint error or code style fix, \`:anger:\` for Solved conflict, \`:recycle:\` for Refactoring, \`:shower:\` for Remove unused features, \`:fire:\` for Remove unnecessary features, \`:pencil2:\` for File name change, \`:file_folder:\` for File move, \`:leftwards_arrow_with_hook:\` for Undo fix, \`:construction:\` for WIP, \`:lock:\` for New feature release range restriction, \`:up:\` for Version up, \`:books:\` for Add or modify documents, \`:memo:\` for Modify comments, \`:green_heart:\` for Modify or improve tests and CI, \`:rocket:\` for Improve performance, \`:cop:\` for Improve security, \`:gear:\` for Change config
prefix は次の中から選択してください.\`fix\` for Bug fixes, \`hotfix\` for Critical bug fixes, \`update\` for Functionality fixes that are not bugs, \`change\` for Functionality fixes due to specification changes, \`add\` for Add new file, \`feat\` for Add new functionality, \`clean\` for Source code cleanup, \`refactor\` for Refactoring, \`style\` for Format fixes, \`disable\` for Disable, \`remove\` for Remove part of code, \`update\` for Functionality fixes that are not bugs, \`rename\` for Rename file, \`move\` for Move file, \`delete\` for Delete file, \`revert\` for Undo fix, \`temp\` for Temporary or work-in-progress commit, \`upgrade\` for Version up, \`docs\` for Documentation fixes, \`test\` for Add test or fix incorrect test, \`perf\` for Fixes that improve performance, \`chore\` for Add or fix build tools or libraries
Subject は英語で簡潔な 30 字程度の要約としてください.
入力例 : チャットのテキストをコピーする機能を追加
返答例 : **Added feature to copy chat text.**\n- \`:+1: update / Added feature to copy text of chats\`\n- \`:sparkles: feat / Add feature to copy chat text\`\n- \`:up: upgrade / Introduce text copy functionality in chat\``;
コメント